Don’t Commit Improperly Formatted Go (golang) Code
Overview
This article will outline how in just a few easy steps using git hooks
how to ensure you never commit improperly formatted code.
I work on a lot of Go (golang) projects, and I still see simple things going wrong. One of them is improperly formatted
Go code. I also include in the category of improperly formatted to mean any code that didn’t use go imports
to sort the import statements.
On the surface, many people might think this is me being a little over-critical of the code quality. In part, that is true. However, the real issue is the fact that it creates unnecessary code-churn in your commits and code diffs. For instance, if I edit a file and make a one line change, but the code wasn’t formatted properly when I got to it, it may result in 10 lines of code changing that have nothing to do with my actual change. This leads to confusion in PR reviews, changes the blame
on a line of code that shouldn’t have changed, etc.
All of this is very avoidable if you are using a technology like git
and employ a hook.
You’ll find a directory in your git project at the root in .git/hooks
. In there will already be some .sample
hooks for you to look at. We are interested in the pre-commit
hook.
This is what my pre-commit hook looks like for all of my Go projects:
#!/usr/bin/env bash
fmtcount=`git ls-files | grep '.go$' | xargs gofmt -l 2>&1 | wc -l`
if [ $fmtcount -gt 0 ]; then
echo "Some files aren't formatted, please run 'go fmt ./...' to format your source code before committing"
exit 1
fi
vetcount=`go vet ./... 2>&1 | wc -l`
if [ $vetcount -gt 0 ]; then
echo "Some files aren't passing vet heuristics, please run 'go vet ./...' to see the errors it flags and correct your source code before committing"
exit 1
fi
Note: I didn’t come up with this pre-commit
file. I learned this trick working with the great developers at Influx Data.
I have this in a gist as well. To install this gist in a pre-commit
hook for your project, run these commands (be sure to be in the root of your project first):
# from the root of your project:
curl -o .git/hooks/pre-commit https://gist.githubusercontent.com/corylanou/3639c901965922d5507ce4acf539de4a/raw/e535b525c408b5b145fed5d17c854c2a6dc90216/pre-commit
chmod +x .git/hooks/pre-commit
And that’s it! Now, the next time you try to commit code that doesn’t pass go fmt
or go vet
you will get an error like this:
$ commit 'test'
Some files aren't formatted, please run 'go fmt ./...' to format your source code before committing
Feel free to contact me with suggestions and feedback on this article on twitter.
More Articles
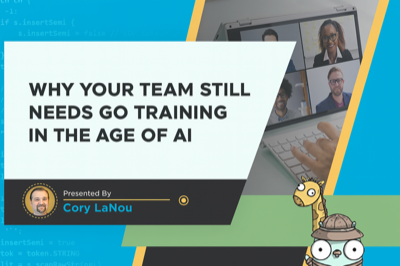
Why Your Team Still Needs Go Training in the Age of AI
Overview
AI hasn't killed software training. In fact, after seeing training demand slow down initially when ChatGPT and Copilot took off, we're now busier than ever. Why? Because teams are discovering what I've been saying all along - AI is only as good as the code it learned from, and most Go code out there isn't idiomatic.
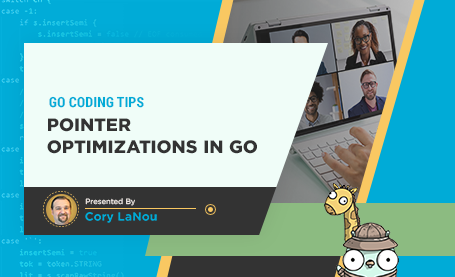
Quick Tips: Pointer Optimizations in Go
Overview
This article explores important performance considerations when working with pointers in Go. We'll cover key topics like returning pointers to local variables, choosing between pointer and value receivers for methods, and how to properly measure and optimize pointer-related performance using Go's built-in tools. Whether you're new to Go or an experienced developer, these tips will help you write more efficient and maintainable code.
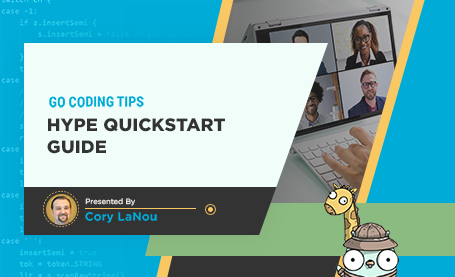
Hype Quick Start Guide
Overview
This article covers the basics of quickly writing a technical article using Hype.